Using the JS runtime, you can set up a Rive animation to track the pointer throughout the viewport.
To make this work, you need to create timelines for each of the extremes of the character's/artwork's directions: Up, Down, Left, and Right. For each timeline set a key for the furthest your art will move in that direction. In the state machine, create 1D blend states that blend between the opposing directions. Set the blend to be controlled by a number input with 0 for the left and down states and 100 for the right and up states.
Optional: create a mid state for each direction, in this case xMid and yMid to control where the art is oriented when the cursor is in the middle of the viewport. This can be helpful if you know your character will be in a position on your page that is not centered in the viewport. Set these values to 50 in the 1D blend.
Here is the way the blend states are set up:
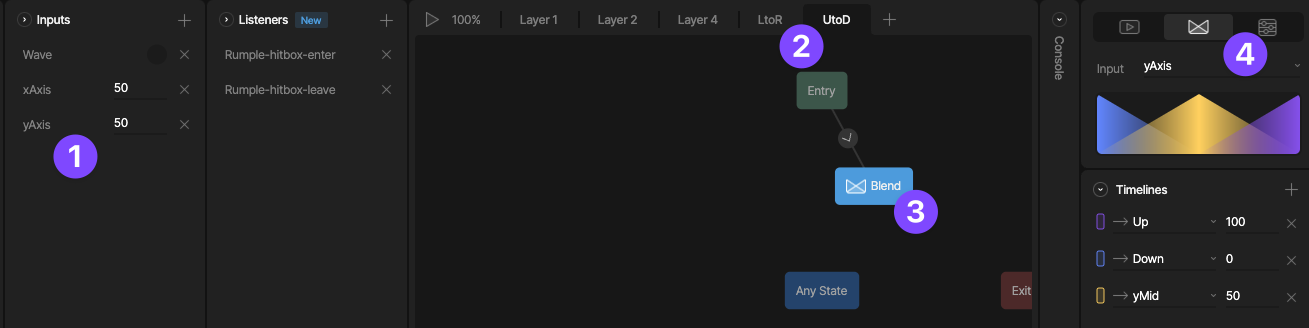
- The xAxis and yAxis are number inputs the drive the blend state and are set by the JS runtime code.
- Create a layer for the Left to Right Blend and another for the Up to Down blend.
- Add a 1D blend state to the state machine.
- Select the corresponding input (yAxis in this screenshot), add the timelines you want to blend and set their blend values.
To set up your JS runtime code, you'll need to know the names of:
- Your .riv file (and it's location)
- The artboard
- The State Machine
- The number inputs used for the blend states (xAxis and yAxis in this case)
Be sure to add the JS runtime script to the <head> tag either for the page you're on or for the whole project:
Here is the code that is used for the embedded example above: